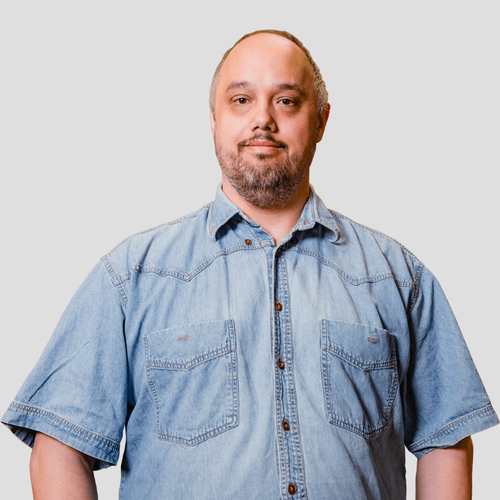
Melvin Louwerse
3 februari 2014
Melvin Louwerse
3 februari 2014
What I skipped in the previous blog because it was dedicated to SOLID was the intro to the workshop.
The tutorial started with a sort introduction of what design patterns actually are.As the tutorial used it so will I, Wikipedia states:
“In software engineering, a design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. A design pattern is not a finished design that can be transformed directly into source or machine code. It is a description or template for how to solve a problem that can be used in many different situations. Patterns are formalized best practices that the programmer must implement in the application.“
A design pattern is a general reusable solution to a commonly occurring problem.
Because it is a solution to a general problem the chances are that you are already using design patterns to some extent. We are all programmers and even if we are all individuals we like to find the best solution. Design patterns are exactly that just the best way written down.
A design pattern is not a finished design that can be transformed directly into source or machine code. Patterns are formalized best practices that the programmer must implement in the application.“
Because design patterns are generic solutions they will seldom be implemented twice the same way because no two projects are the same they will not require the exact same solution. That is the strength of design patterns they give a common ground and even if the code is not the same the structure will be. Its a tool to help you make the correct choices.
The decorator parrot makes sure that it is easy to add dynamic functionality to a class. It adds much value when creating a class for each of the needed (combination of)functionality would result in much classes. In the example a print statement was used to demonstrate how this pattern works.
class Print {
public function print($string)
{
print($text);
}
}
Take for example the string “test” and you wish to strip all the HTML tags from that string before printing, print it bold or do both. Doing this by creating a class for each of these would result in 4 classes (original included) which would be print, printStriptags, printBold andPrintBoldStripTags.
Now we rewrite this to use the Decorator pattern:
class PrintStripTags extends Print{
protected $printer = null;
public function \_\_constuct(Print $printer) {
$this->printer = $printer;
}
public function print($string)
{
ob\_start();
$this->printer->print($text);
$string = $ob\_get\_contents();
ob\_end\_clean();
print(strip\_tags($text));
}
}
class PrintBold extends Print{
protected $printer = null;
public function \_\_constuct(Print $printer)
{
$this->printer = $printer;
}
public function print($string)
{
ob\_start();
$this->printer->print($text);
$string = $ob\_get\_contents();
ob\_end\_clean();
print(“**” . $text . “**”);
}
}
new PrintBold(new Print())->print('test'); ==> **test
**new PrintStripTags(new Print())->print('test'); ==> test
new PrintBold(new PrintStripTags(new Print())->print('test'); ==> **test******
As you can see we need one class less. Of course one class less does not seem like a big achievement. But what if we want to add new functionality to the mix, lets say we want to print ithe text camel cased.
Without the decorator pattern we would suddenly need 12 classes to support all variations while with the decorator pattern it would be just add one extra class making the total of 5 classes.
Maybe you read my last blog about SOLID and think “Does this not break the open/closed principle? You change how the constructor looks for each class.”.
And yes you could say the interface is changed and because in the print class we do not have a constructor. The other classes do have a constructor and we even have to pass an object to it.
However the speaker noted during the tutorial that the magic methods of PHP like __construct are not seen as part of the interface, and in that case we would not break SOLID.
The mediator pattern describes how classes should communicate with each-other to have loose coupling. The best way to explain this is to use some illustrations. As you can see in the first image there are 5 classes that have to communicate with each other class resulting in 4 links to an other class and this for every class. So each class has to know about 4 other classes. In the worse case scenario a change in one of those classes would break 4 others.
Now we create the same situation but using the mediator pattern. To realize this we would have to add one extra class (the mediator) this class will take charge of the communications between the other classes. And as you can see in the next image the paths of communication are suddenly much more clear and readable , Now when something changes you should only change code in the mediator itself.
The singleton pattern makes sure that its not possible to create more then one instance of an object. This can be important in some cases. A db is a good example for this, you don’t want that more then one connection to the same db is opened it could totally mess-up your transactions. Ans since you don’t want that the singleton could be a viable option to enforce that 1 and only one connection is opened.
The following code illustrates how a singleton is created in PHP.
class Db {
private static $instance;
private function \_\_construct()
{
}
private function \_\_clone()
{
}
public static function getInstance()
{
if (empty(self::$instance)) {
self:$instance = new Db();
}
return self::instance;
}
}
This is the minimum of code necessary to create a singleton. Because the constructor is private it is not possible to create an instance of the class externally. To get the instance you have to call the getInstance on the class. Getinstance will then check if it already has created an instance for you and if it has it will return it, else it will create a new one store it in a variable and return it to you.
However the singleton is a bit the odd man out in pattern land as it breaks SOLID.
The “single responsibility principle” states that every class should do only one thing. But if you make a singleton that is impossible if you actually want your singleton to do something take the database as example again we want to make a database connection otherwise the class serves no purpose but it would mean that the class is responsible for creating an instance and creating a connection which are different responsibilities.
Testing the singleton is also not possible as it will always give only one instance back and is not mockable(is createable in only one way)
Often its wiser to use dependency injection instead of the singleton pattern.
The “Adapter pattern” is closely connect to the “Interface segregation” and “Dependency inversion” principles. The main functions of this pattern are to provide a simple interface for not complex functionality and to provide a uniform interface for usage within your code. Usually this is used when you have no control over the interface so its an extra layer between your own code and the functionality a library offers.
Also here the database will serve as a good example.
Let start with adding connecting to a MySQL database from the controller (this is all purely to illustrate and is not actual code of course). For the sake of the explanation lets say we make the connection like this and then get the results.
$db = new Db();
$connection = $db->connect();
$connection->maxResults(1);
$results = $connection->getResults();
Everything works, your connection is created and you get the expected results. But now something changes for one reason or the other you will have to change to a MsSql database now everywhere you used the database you have to change your code to something like this.
$db = new MsDb(); $connection = $db->makeConnection(); $results = $connection->getQuery()->getResults(1);
Now you have a problem everywhere that code is used you will have to change it and every variation of that code that is probably more complex will have to be changed as well.
The adapter pattern would have saved a lot of time and crunching of teeth here. If we set up our code from the start as in the example that follows we would have to change our database logic in only one place.
interface iDb
{
protected $connection;
public function setMax($max);
public function getResults();
}
class mysqlDb implements iDb
{
protected $connection;
public function \_\_construct()
{
$db = new Db();
$this->connection = $db->connect();
}
public function setMax($max)
{
$connection->setMax($max);
}
public function getResults()
{
return $connection->getResults();
}
}
class mssqlDb implements iDb
{
protected $connection;
protected $max;
public function \_\_construct()
{
$db = new MsDb();
$this->connection = $db->makeConnection();
}
public function setMax($max)
{
$this->max = $max;
}
public function getResults()
{
return $connection->getQuery()->getResults($this->max);
}
}
In the controller you would then use the msSqlDb or mySqDbl classes that have the same interface and are interchangeable(Liskov substitution principle. And even if in the msSql example you have to do more to get the same result the Adapter handles that for you and the controller won’t have to know of this.
At a first glance the “facade pattern” looks a lot like the “adapter pattern” however there is one important difference, the facade offers an interface to a collection of functionality where the adapter offers a interface to a single class.
A good example here would be modifying a JPEG file, say you want to make the image smaller then convert it to a gray scale and after that convert it to a PNG file. If you have a library for this there would be different functions and most likely different classes (or maybe you like the convert to gray scale from a totally different library more) classes that handle these transformations.
But you want to do these transformations in different places in your own code and of course you don’t want to have to put the same code everywhere in your application.
Without the facade you would maybe have something like in the example.
Class uploadFile
{
public function convert($file)
{
$resizer = new Resizer\_library();
$converter = new Converter\_library()
$resizedFile = $resizer->resize(10 , 10, $file);
$pngFile = $converter->jpegToPng($resizedFile);
$grayFile = $converter->toGrayScale($pngFile);
return $grayFile
}
}
class importFile
{
public function convert($file)
{
$resizer = new Resizer\_library();
$converter = new Converter\_library()
$resizedFile = $resizer->resize(10 , 10, $file);
$pngFile = $converter->jpegToPng($resizedFile);
$grayFile = $converter->toGrayScale($pngFile);
return $grayFile
}
}
However when you use the facade pattern you will get.
class converterFacade
{
public function convert($file)
{
$resizer = new Resizer\_library();
$converter = new Converter\_library()
$resizedFile = $resizer->resize(10 , 10, $file);
$pngFile = $converter->jpegToPng($resizedFile);
$grayFile = $converter->toGrayScale($pngFile);
return $grayFile
}
}
class uploadFile
{
public function convert($file)
{
$converter = new ConverterFacade();
$file = $convert($file);
}
}
class importFile
{
public function convert($file)
{
$converter = new ConverterFacade();
$file = $convert($file);
}
}
Like this you did not only limit your code duplication but you also created a simpler interface to the functionality that you need. Everywhere that you want to do the same conversion you just have to know of your facade class instead of all the separate functions and classes. It also makes it easier to change functionality if you want to convert to a GIF image instead of a PNG you have to change this in only one place and the rest of your code will work without any problem.
The “chain of responsibility” pattern describes that classes should only handle commands they know(their responsibility) If they cannot handle the responsibility they will pass it to the next in the chain that will check if it is his responsibility and so creating a chain(hence the name chain of responsibility). If the command reaches the end of the chain without being handled it usually means the chain was not configured good.
The example that was given during the tutorial gave as example a router.
Imagine you have 3 classes where in the following order it does the next checks.
Now we visit www.test.com/index it will follow the chain, step 1 will not know what to do with it because index is not an admin url, step 2 will then check but this is also not a profile page and finally step 1 will recognize the url..
But in the same example if we visit www.test.com/cart it will follow the same route only at step 3 it is not recognized either so it will throw an error normally this would mean there is an error however In case of a router its perfectly reasonable somebody types a wrong address so in that case when not found in step 3 it could throw a 404 not found error.
The last pattern that was demonstrated was the “Observer pattern”. This pattern describes how different objects can subscribe themselves to be notified of a change and so effectively observe that object. To realize this the object that is observable has a function that the observers can subscribe themselves, the observers themselves all inherit from the same abstract class so the observer knows what interface it can use to communicate its changes to. And of course because its all the same interface the observer doesn’t have to know what specific objects are observing(Liskov substitution principle)
abstract class Observer
{
abstract public function update();
}
class EmailObserver extends Observer
{
public function update(iObservable $subject)
{
$message = 'Hello page ” . $subject->getPageName() . ' was observed ';
$mail = new Mailer(“SomeMail@SomeMail.com”);
$mailer->send($message);
}
}
class CacheRefreshObserver extends Observer
{
public function update(iObservable $subject)
{
$this->clearCache($subject->getUrl());
}
}
class GoogleNotifyObserver extends Observer
{
public function update(iObservable $subject)
{
$connector = new GoogleConnector();
$connector->notifyGoogle($subject->getUrl());
}
}
class Page implements iObservable
{
protected $observers = array();
public function attach(Observer $observer)
{
$this->observers\[\] =$observer;
}
protected function notify()
{
foreach ($this->observers as $observer) {
$observer->notify($this);
}
}
public function save($data)
{
$this->saveToDb($data)
$this->notify();
}
}
//somewhere in the code
$page = new Page();
$page->attach(new EmailObserver());
$page->attach(new GoogleNotifyObserver()):
$page->attach(new CacheRefreshObserver());
Now when a pages is saved in the example the observers will take care of sending the mail, clear the cache and notify google to index the page. And all this without that the observed class has to know anything about it all logic is handled by the observers and the observed class can do what it does best and that is saving pages.
During the tutorial a handy resources was noted: https://github.com/domnikl/DesignPatternsPHP
This is a project that gives a short description of most design patterns with PHP code examples to accompany them.