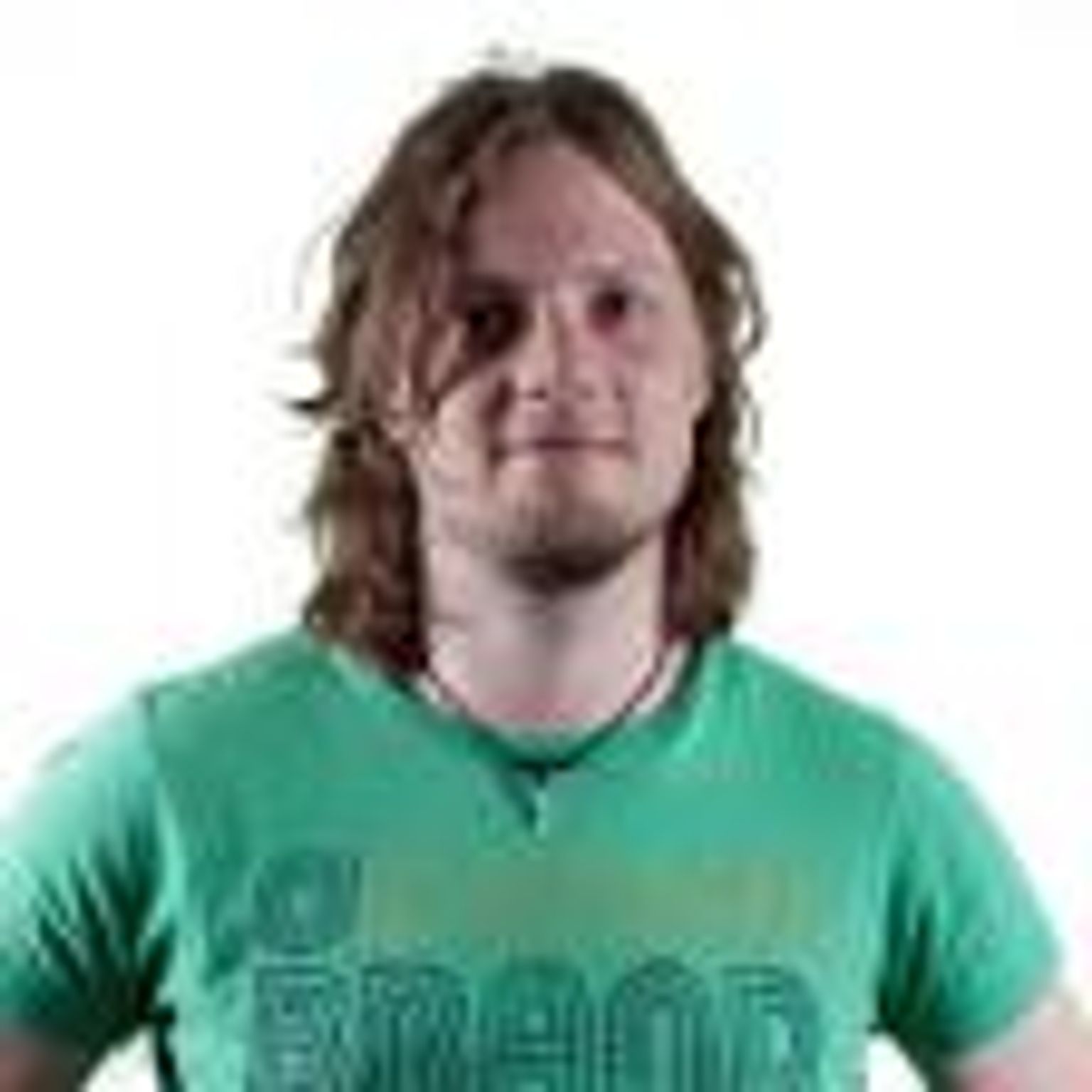
Scato Eggen
30 oktober 2015
Scato Eggen
30 oktober 2015
Using a style guide helps keep code more readable, which makes it more maintainable. It can also prevent you from introducing bugs which can be hard to spot (by making semicolons mandatory for example). Enforcing code styles is hard. You can use code reviews to do so, but luckily, there are some tools to help you.
This blog post tells you how to enforce the Airbnb Style Guide using ESLint and Ant.
There are a lot of style guides to choose from. You could even write your own style guide. I recommend the Airbnb Style Guide. It includes a section on ECMAScript 6 and has default configurations for all major code style tools.
The only downside of the Airbnb Style Guide is that it requires 2 spaces of indentation. In the example below, I will change this to 4 spaces, since this is what we use for PHP.
There are a number of tools to help you enforce code style, but I think ESLint is the best one. It checks for possible bugs (like JSLint) as well as whitespace (like JSCS). It even supports JSX!
Let’s get started. We can install ESLint and the Airbnb configuration in one go:
npm install --save-dev eslint-config-airbnb babel-eslint eslint-plugin-react eslint
Next, we need to create a config file named .eslintrc in the root folder of the project, which contains the following:
{
"extends": "airbnb",
"rules": {
"indent": [2, 4],
"id-length": [2, {"exceptions": ["$", "_"]}]
}
}
Here, we overrule the indentation by saying it’s still an error (2), but the size should be 4 (not 2). On top of that, we add two exceptions for short identifiers, so we can use jQuery and Underscore in a more convenient way.
To run ESLint, simply enter the following command in your terminal:
node_modules/.bin/eslint
The next step is to run ESLint as part of our continuous integration. The way to do this depends entirely on the way you do continuous integration.
Usually, we use our Ibuildings QA Tools. This uses Ant to run all the tests, so we just add a target to build.xml.
There is a separate Ant script for the pre-commit hook, which checks only files that have been changed:
Don’t forget to change the source directories and add the targets as dependencies of the main target.
When following the Airbnb Style Guide, there are some errors that may seem strange at first. It turns out these have to do with ECMAScript 6.
For one, strict mode is not permitted. If you transpile your code with Babel, strict mode is added automatically, so there’s no need to add it yourself.
Also, the var keyword is not allowed. ECMAScript 6 adds the keywords let and const that behave differently. You should use these instead.
Finally, anonymous functions have to be named. This might be strange, but the Airbnb Style Guide states that you should use the fat arrow syntax (=>), unless a function is very complex, in which case it should be named.
If you are not using ECMAScript 6, you can suppress these errors by adding the following rules to .eslintrc:
{
"rules": {
"strict": 0,
"no-var": 0,
"func-names": 0
}
}
A zero means that it is not an error (2), nor a warning (1).
Checkout the following links if you want to know more.